A variable is just a cup. It has a size, and it can hold something.
In Java, cups come in two main styles: primitive and reference.
In Java, cups come in two main styles: primitive and reference.
Primitive cups hold primitive values.
Reference cups hold remote controls to objects.
Reference cups hold remote controls to objects.
Primitives:
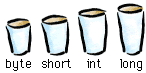
In Java, each of these cups (float, char, long, etc.) is a specific size. Byte is the smallest, double and long are the largest. Rather than measure in milliliters (or ounces as we do in the US) Java variables have a size measured in bits:
byte - 8 bits
short - 16 bits
int - 32 bits
long - 64 bits
short - 16 bits
int - 32 bits
long - 64 bits
All of these integer types are SIGNED. The leftmost bit represents the sign (positive or negative) and is NOT part of the value. So with a byte, for instance, you don't get the whole 8 bits to represent your value. You get 7. This gives you a range, for bytes, of :
(-2 to the 7th) through (2 to the 7th) -1. Why that little -1 on the end? Because zero is in there, and zero counts as negative. Works the same way with the others.
(-2 to the 7th) through (2 to the 7th) -1. Why that little -1 on the end? Because zero is in there, and zero counts as negative. Works the same way with the others.
float - 32 bits
double - 64 bits
double - 64 bits
References:
In Java, if you want to stick an object in a variable, remember that the object is created out on the garbage-collectible heap. Always. So it's not IN the variable. There aren't giant, expandable cups which can be made big enough to hold any object. And unlike C/C++, there aren't cups which hold the exact memory location of the object.
In Java, objects are created on the heap, and a REFERENCE to the object is stored in the cup. Think of it as a remote control to a specific type of object.
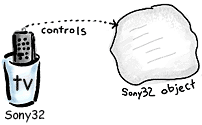
In Java you say:
Sony32 tv; // declare but don't initialize with an actual Sony32 object.
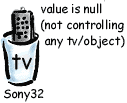
In Java, remote controls are called references. They store a value which the Java Virtual Machine (JVM) uses to get to your object. It sure looks and feels a lot like a pointer, and it might very well be a pointer to a pointer, or...
You Can't Know. It's an implementation detail that you, as a programmer, can't access. Don't even think about it. There's no way to use that value other than to access the methods and variables of the actual object the reference refers to. That's part of what makes Java safer than C/C++. You can not go directly to any arbitrary memory location. The JVM allocates memory on your behalf, for your object, and stores an address-like thing in the reference cup (which is most likely a 32-bit cup, but not guaranteed to be).
Now into the topic of discussion "Pass By Value"
1) int x = 3;
2) int y = x;
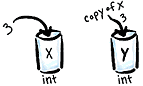
In line 1, a cup called x, of size int, is created and given the value 3.
In line 2, a cup called y, of size int, is created and given the value... 3.
The x variable is not affected!
Java COPIES the value of x (which is 3) and puts that COPY into y.
This is PASS-BY-VALUE. Which you can think of as PASS-BY-COPY. The value is copied, and that's what gets shoved into the new cup. You don't stuff one cup into another one.
Saying int y = x does NOT mean "put the x cup into y". It means "copy the value inside x and put that copy into y".
So... what about Reference Variables (remote controls)? How does THAT work?
Not so tricky, in fact the rule is the same.
Not so tricky, in fact the rule is the same.
References do the same thing. You get a copy of the reference.
So if I say:
Cat A = new Cat();
Cat B = A;
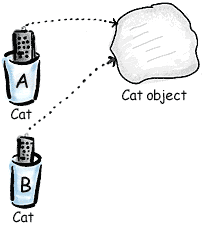
The remote control in A is copied. Not the object it refers to.
You've still got just one Cat object.But now you have two different references (remote controls) controlling the same Cat object.
You've still got just one Cat object.But now you have two different references (remote controls) controlling the same Cat object.
Cat A = new Cat();
doStuff(A);
doStuff(A);
void doStuff(Cat B) {
B = new Cat(); //did NOT affect the A reference
}
}
Doing this simply "points" B to control a different object. A is still happy.
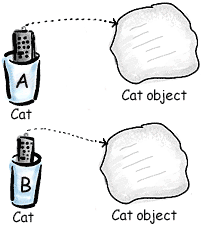
So repeat after me:
Java is pass-by-value.
(OK, once again... with feeling.)
Java is pass-by-value.
For primitives, you pass a copy of the actual value.
For references to objects, you pass a copy of the reference (the remote control).
You never pass the object. All objects are stored on the heap. Always.
Now go have an extra big cup of coffee and write some code.
References:
1. http://www.javaranch.com/campfire/StoryCups.jsp2. http://www.javaranch.com/campfire/StoryPassBy.jsp
Comments
Post a Comment